> Queue Acceptor
The Queue Acceptor is a tool used to accept League of Legends queues (get into a game) while away from the computer. Whether I wanted to take a quick trip to the bathroom, grab a drink of water, or was just feeling lazy, I can boot up this tool to get into game hands free (for people not familiar with League of Legends, click here for video example before reading). This tool went through many different iterations. When I was first learning to code, I was hesitant to use a traditional language to create this, so I resorted to utilizing AutoHotkey (AHK). AutoHotkey allows for creating macros to automate tasks. The script went as follows:

AHK Queue Acceptor
> Line 1 - Bring the League of Legends Window to the forefront of your window (active window)
> Line 2 & 3 - set the delay between Key and Mouse inputs to 0ms (-1 denotes no delay).
> Line 4 & 5 - set the coordinate mode for pixel searchers and mouse commands to be relative to the size of the entire screen (1920x1090) as opposed to an individual window.
> Line 7 - Pop-up message box to prompt the user that he application has started.
> Line 9 - Denotes the image that will be searched for later in the script (the "ACCEPT!" button).
​
​
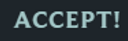
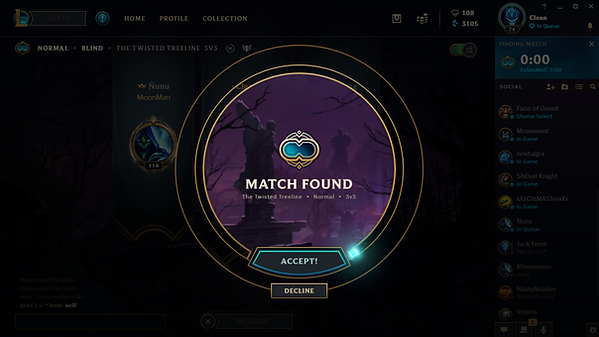
Match Found Window
Image Being Searched For
> Line 12 - Set the client size to default (1280x720).
> Line 14 - Begin Loop to recursively search for the image above (meaning a match has been found).
> Line 16 - Check entire screen for image match.
> Line 17 - If image is found, then:
> Line 19 - Store coordinates of where mouse currently is (at the time of match being found).
> Line 20 - Click the location of the image that as found (the "ACCEPT!" button).
> Line 21 - Move mouse back to where it was prior to clicking "ACCEPT!".
* Note - Lines 19 and 21 is used in cases where you are at your computer, so the user will not feel their mouse movements being interrupted if the queue pops (mouse returns back to where user left it).
> Line 25 - Set a macro for the "Esc" key:
> Line 26 - Delete the image stored previously (see above).
> Line 27- Exit the app.
​
Despite the Queue Acceptor being fully functional in its AHK versioning, I was not entirely satisfied with this approach, as it felt like I had "cheated" myself out of an experience to dive deeper into coding in a more suitable and career-applicable language. This was created during my first semester of college, and during this time I was enrolled in my first Computer Science course, which happened to be focused around Python, so I decided this would be a logical next step forward.
AHK to Python
As I quickly found out, creating this program in Python would be a whole lot more work than AHK. AHK did not require any true interaction with the back-end of the League Client, and solely operated by scanning the screen and moving the mouse. Utilizing Python however required me to discover the API calls that made the client operate. This was not public information, and being an entry-level programmer, required more work than I had anticipated, and required me to get very creative. With most programming problems I encounter, there tends to always be a "solution" online, and I was just a Google search away from finding the info that I needed. However, the League of Legends client was by and large uncharted waters, and there was no public info online that I could find to help me, so I was on my own.
I began researching APIs, what they were, and how they worked, and this helped me narrow down what I needed to do to make this application a reality. Using a software called Fiddler, I was able to isolate and intercept the API calls needed to create scripts that interacted with the client., and using Postman I could test these calls and their functions.
​
Despite now having access to the League Client API calls, I quickly learned that just having the endpoints would not be enough. I discovered that each time you opened a new instance of the League client, the API call would slightly change in two places: the port and the Basic token.
​
After creating a second instance of the League of Legends folder, I was able to compare the files that existed when the client was open, and when the client was closed. Upon doing this, I discovered a file named "lockfile", and it was in this file that the two missing links to a successful API call were located.
​
​
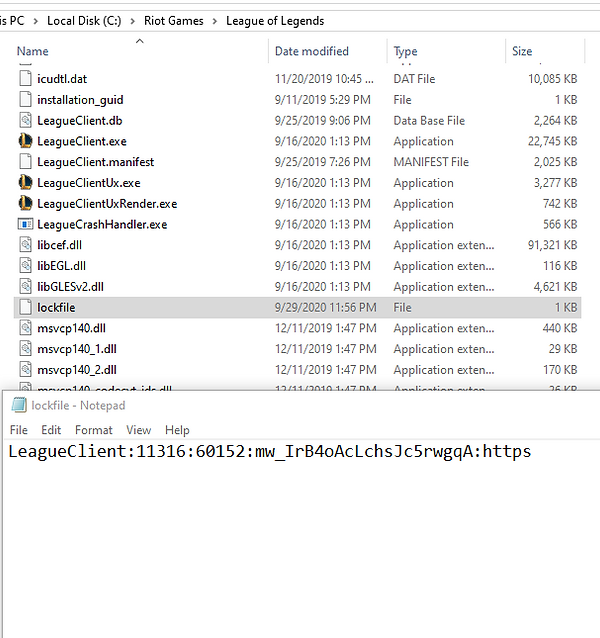
lockfile within League of Legends folder
In the image above, you can see what appears to be a seemingly random sequence of strings. However for the sake of this project, all I cared about was the third and fourth strings. The third string "60152" is the unique port identifier to the League Client instance that i opened for this screenshot and has to be inserted into the API URL. The 4th string (in this instance, "mw_IrB4oAclchsJc5rwgqA") is whats called the Basic Authentication Token, which is a Base64 encoded string which authorizes the HTTPS request, and is passed in as a Header, with key of "Authorization" and a Value of "Basic + token". However, in its current state, this string is in UTF-8, and must be encoded into Base64 for it to be passed as a Basic Authentication token. For the sake of Riot's API, the string "riot:" must be appended to the beginning of the UTF-8 string, and then the resulting string can be encoded into Base64 to be used as a Header.
​
​
​
​
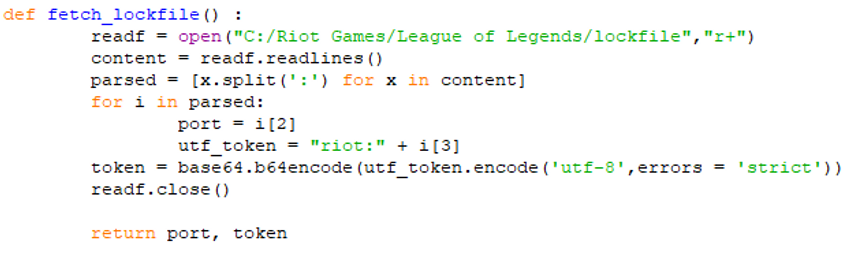
Getting port and Basic token from lockfile
The code above is the first function in the Python version of the Queue Acceptor, and must be executed before any API calls are used. It reads as follows:
​
> Line 1: Defining the function "fetch_lockfile()" which will be called later.
> Line 2: Opening a text stream for the contents of the lockfile into the variable "readf".
> Line 3: Setting the contents of the text stream into the variable "content".
> Line 4: Parsing the 5 strings within the lockfile.
> Line 5: Iterate through the parsed strings:
> Line 6: Set the variable "port" equal to the contents of the third string in the "parsed" variable.
> Line 7: Set the variable "utf_token" to the string "riot:" + the fourth string in the "parsed" variable.
> Line 8: Encode the utf_token variable created above into Base64 (to be used as the Basic token)
> Line 10: Close the text stream created above.
> Line 12: Return the port and token variables to be used in other functions.
After the above code is ran, and the port and token variables are obtained, I was now able to begin coding specific purpose of this project. The above code can and must be used for ANY project relating to the League of Legends client API, and will be used in later projects I worked with.
To give some background, to play a game of League of Legends, a player first must go through these three steps:
1) Click the Play button and select the game mode they would like to play.
2) Queue Up/Find A Match(a fancy way of saying "begin searching for other players to play with")
3) Once players are found, accept the queue (let the game know you are ready to play)
​
The purpose of my program was to automate this third step, allowing you to step away from your computer and do whatever you wanted to do while you waited for your game to be ready.
Now that you understand the process of getting into a game, let's get back into some code:
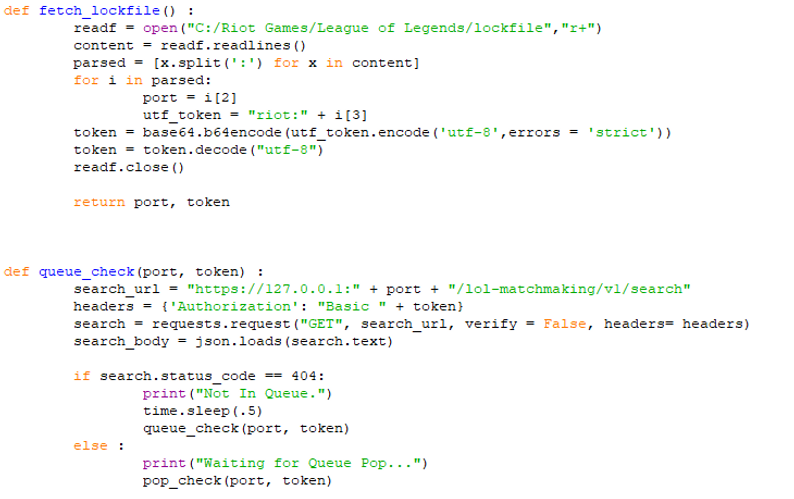
"In Queue" status check
In the image above, the first chunk of code is the same as the one discussed earlier. The second chunk, is a method of checking to see if the user is actively in a queue. The reason being is because if they aren't in a queue, then the script has no purpose, because in the end, it's only job is to accept a queue. An API call is used, which will return a 404 error if the user is not in a queue. However if we do not see this error, we know the player is actively looking for a game, and we can move onto the next part of the code as we actively wait for a game to be found.
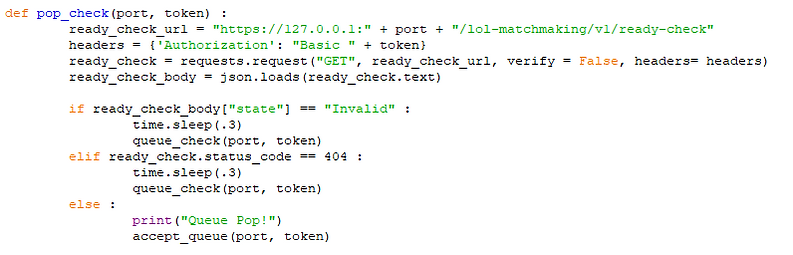
"Queue Pop" status check
In League of Legends lingo, a "Queue Pop" means a game has been found after waiting in your queue. It is now up to a player to click the Accept button. So, in the above code, we are checking to see if a game has been found. Once again, we are using one of the API calls I acquired to do just that. The API call is used, and we are checking for two fail cases, one being the user is not in queue anymore or if a game has not yet been found ( no queue pop). However if neither of these are the case, that means we have found a game and are ready to accept the queue, which brings us to our final piece of code.

Accept the queue
To give some context, our previous two API calls above have been what are called GET methods. These are used to receive information from the server. In my case, I was performing status checks using the information that was given to me. For this last chunk of code however, I am using the POST method. which is used to send data to the server. In this case, I am using Python's requests library to send a the information back to the server saying I am accepting the queue.
​
The entire process can be summarized as follows:
1) Check if user is in queue (GET method)
2) Check if a game has been found (GET method)
3) If a game has been found, accept the queue (POST method)
​
The first two are requesting information, while the third is sending information back. I know this is a lot of tech jargon, but hopefully I was able to break it down in a way that made sense.
​
Here is the finished product. You will see the script running in the command line above spelling out what , while the client is below with the automated functions being performed:
Video Demonstration
1) The script recognizes the user is not currently in queue and gives the "Not In Queue" status update.
2) The user clicked "Find Match", causing the script to recognize the user is in queue. The script is now waiting for a match to be found ("Waiting for Queue Pop..."), or for the user to exit the queue.
3) The user exits the queue, and the script updates and displays "Queue Cancelled" and returns back to the "Not In Queue" status, awaiting further user input.
4) The user has clicked "Find Match" again (repeating step 2 above).
5) The queue pops (a match is found), and the script instantly uses the API call to accept the queue.
​
Wait... One More Thing!
It was at this point I realized that my original goal of being able to leave my computer while in a queue was only half-doable. What if I got up, left my room, and the queue popped without me knowing? This is when I decided I would have the script text me when the queue popped! That way, I could bring my phone with me downstairs or to the bathroom and get notified when a game was found, so I knew to come back to my computer as soon as possible. This made the program a lot more practical and gave me peace of mind when I left my computer.
​
I'm not going to get deep into the code for this part (I actually switched over to Node.js and used Twilio to make this possible), but essentially if a game was found, I would get a text message within ~2 seconds notifying me. At the time of writing this, I am currently coding a way to be able to select my character and do more functions via texting back from my phone which is an entirely new mountain to climb, but for now this is the end!
